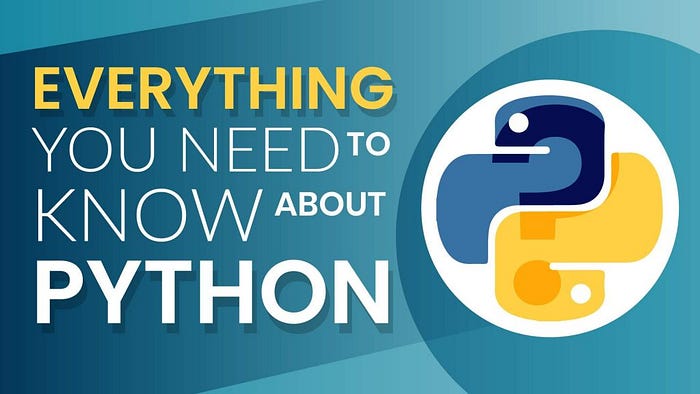
- Which is NOT a characteristic of namedtuples?
A) Each member of a namedtuple object can be indexed to directly, just like in a regular tuple.
B) You can assign a name to each of the namedtuple members and refer to them that way, similar to how you would access keys in a dictionary.
C) No import is needed to use namedtuples because they are available in the standard library.
D) namedtuples are just as memory efficient as regular tuples.
Answer: C (To use namedtuple function we need to import the collections module. It accepts immutable objects just like regular tuples, dictionary keys, or set elements. In fact it functions just like a regular tuple except with more naming benefits.)
2. What happens when you use the built-in function any() on a list?
A) The any() function returns a Boolean value that answers the question “Are there any items in the list?”
B) The any() function will randomly return any item from the list.
C) The any() function returns True if any item in the list evaluates to True. Otherwise, it returns False.
D) The any() function takes as arguments the list to check inside, and the item to check for. If “any” of the items in the list match the item to check for, the function returns True.
Answer: C
3. What is the algorithmic paradigm of merge sort?
A) decrease and conquer
B) backtracking
C) dynamic programming
D) divide and conquer
Answer: D (Merge sort basically breaks down a list into single elements, then compare and merge every 1,2,4,8… adjacent elements sortedly and recursively)
4. Which of the following is true about how numeric data would be or ganized in a binary search tree?
A) For any given node in a binary search tree, the child node to its left is less than the value of the given node and the child node to its right is greater than the given node.
B) The smallest numeric value would go in the top-most node. The next highest number would go in its left child node, then the next highest number after that would go in its right child node. This pattern would continue until all numeric values were in their own node.
C) The top node of a library search tree would be an arbitrary number. All the nodes on the left of the top node need to be less than the top node’s number, but they don’t need to be ordered in any particular way.
D) Binary search tree cannot be used to organize and search through numeric data, given the complications that arise with very deep trees.
Answer: A (The point of a binary search tree is to have all the nodes in sequential order from left to right. Therefore we can apply the divide and conquer algorithm like bisecting)
5. def print_alpha_nums(abc_list, num_list):
…….for char in abc_list:
………..for num in num_list:
………..print(char, num)
…….return
print_alpha_nums([‘a’, ‘b’, ‘c’], [1, 2, 3])
A)
a 1 2 3
b 1 2 3
c 1 2 3
B)
aaa
bbb
ccc
111
222
333
C)
a 1
a 2
a 3
b 1
b 2
b 3
c 1
c 2
c 3
D)
[‘a’, ‘b’, ‘c’], [1, 2, 3]
Answer: C (For nested for loops, always remember the inner most loop will be executed first)
6. What is one of the most common uses of Python’s sys library?
A) to scan the health of your Python ecosystem while inside a virutal environment
B) to capture command-line arguments given at a file’s runtime
C) to take a snapshot of all the packages and libraries in your virtual environment
D) to connect various systems, such as connecting a web front end, an API service, a database, and a mobile app
Answer: B
Type: module
String form: <module 'sys' (built-in)>
Docstring:
This module provides access to some objects used or maintained by the
interpreter and to functions that interact strongly with the interpreter.Dynamic objects:argv -- command line arguments; argv[0] is the script pathname if known
path -- module search path; path[0] is the script directory, else ''
modules -- dictionary of loaded modulesdisplayhook -- called to show results in an interactive session
excepthook -- called to handle any uncaught exception other than SystemExit
To customize printing in an interactive session or to install a custom
top-level exception handler, assign other functions to replace these.stdin -- standard input file object; used by input()
stdout -- standard output file object; used by print()
stderr -- standard error object; used for error messages
By assigning other file objects (or objects that behave like files)
to these, it is possible to redirect all of the interpreter's I/O.last_type -- type of last uncaught exception
last_value -- value of last uncaught exception
last_traceback -- traceback of last uncaught exception
These three are only available in an interactive session after a
traceback has been printed.Static objects:builtin_module_names -- tuple of module names built into this interpreter
copyright -- copyright notice pertaining to this interpreter
exec_prefix -- prefix used to find the machine-specific Python library
executable -- absolute path of the executable binary of the Python interpreter
float_info -- a struct sequence with information about the float implementation.
float_repr_style -- string indicating the style of repr() output for floats
hash_info -- a struct sequence with information about the hash algorithm.
hexversion -- version information encoded as a single integer
implementation -- Python implementation information.
int_info -- a struct sequence with information about the int implementation.
maxsize -- the largest supported length of containers.
maxunicode -- the value of the largest Unicode code point
platform -- platform identifier
prefix -- prefix used to find the Python library
thread_info -- a struct sequence with information about the thread implementation.
version -- the version of this interpreter as a string
version_info -- version information as a named tuple
__stdin__ -- the original stdin; don't touch!
__stdout__ -- the original stdout; don't touch!
__stderr__ -- the original stderr; don't touch!
__displayhook__ -- the original displayhook; don't touch!
__excepthook__ -- the original excepthook; don't touch!Functions:displayhook() -- print an object to the screen, and save it in builtins._
excepthook() -- print an exception and its traceback to sys.stderr
exc_info() -- return thread-safe information about the current exception
exit() -- exit the interpreter by raising SystemExit
getdlopenflags() -- returns flags to be used for dlopen() calls
getprofile() -- get the global profiling function
getrefcount() -- return the reference count for an object (plus one :-)
getrecursionlimit() -- return the max recursion depth for the interpreter
getsizeof() -- return the size of an object in bytes
gettrace() -- get the global debug tracing function
setcheckinterval() -- control how often the interpreter checks for events
setdlopenflags() -- set the flags to be used for dlopen() calls
setprofile() -- set the global profiling function
setrecursionlimit() -- set the max recursion depth for the interpreter
settrace() -- set the global debug tracing function
7. What built-in list method would you use to remove items from a list?
A) .delete()
B) del(my_list)
C) pop(my_list)
D) .pop()
Answer: D (list.pop() is for removing by index and list.remove() is for removing by value. Note del is a python built-in keyword to delete dictionary keys, but there is no such .delete() method in the built-in modules)
8. What is an instance method?
A) An instance method is a regular function that belongs to a class, but it must return None.
B) An instance method is any class method that doesn’t take any arguments.
C) Instance methods hold data related to the instance
D) Instance methods can modify the state of an instance or the state of the parent class
Answer: C (Instance method can only access data unique to that specific instance, which is the most common method in a class rather than static and class method without the need of decorator. An instance method behaves like a regular function, but must have self as its argument)
9. What is the runtime complexity of accessing a value in a dictionary by using its key?
A) O(1), also called constant time
B) O(n), also called linear time
C) O(log, n), also called logarithmic time
D) O(n²), also called quadratic time
Answer: A (According to Big O algorithm runtime notation, for dictionary using hash tables, we will always try to find the hashable key, which results just one value. Hence O(1). For example, list look-up is less efficient than dictionaries because of O(n) for reading the whole list with length n. On the other hand, binary search has O(log, n), which is also described in question #3 above.)
10. Which statement does NOT describe the object-oriented programming concept of encapsulation?
A) It keeps data and the methods that can manipulate that data in one place.
B) It only allows the data to be changed by methods.
C) It protects the data from outside interference.
D) A parent class is encapsulated and no data from the parent class passes on to the child class.
Answer: D (D is true. It talks about class inheritance. One of the points of encapsulation is to avoid variable overriding. For example, we use “__foo” (= _classname__foo) in a class to define a super private variable, which cannot be called outside of a class or be inherited. And we use “_foo” to define a semi-private variable in a class which can inherit and be called outside of class. The underscore is for distinguishing with the public variables. One common tip is that if you use too many “global”s in a function to define variables, then it may indicate OOP.)
***Thanks for reading! Any questions or suggestions you are welcome to chat me on the wechat subscription account: gnu_isnot_unix